TI Emulation, Functions in Brass and Gemini on the Sega Game Gear
Tuesday, 13th February 2007
This post got me wondering about a TI emulator. I'd rather finish the SMS one first, but so as to provide some pictures for this journal I wrote a T6A04 emulator (to you and me, that's the LCD display driver chip in the TI-82/83 series calculators). In all, it's less than a hundred lines of code.
The problem with TI emulation is that one needs to emulate the TIOS to be able to do anything meaningful. Alas, I had zero documentation on the memory layout of the TI calculators, and couldn't really shoe-horn the ROM dump into a 64KB RAM, so left it out entirely. That limits my options as to what I can show, but here's my Microhertz demo -


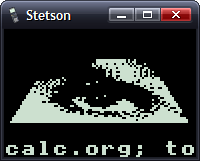


I've added native support for functions in Brass.
The old Brass could do some function-type things using directives; for example, compare the two source files here:
.fopen fhnd, "test.txt" ; Opens 'test.txt' and stores a handle in fhnd .fsize fhnd, test_size ; Stores the size of the file in test_size .for i, 1, test_size .fread fhnd, chr ; Read a byte and store it as "chr" .if chr >= 'a' && chr <= 'z' ; Is it a lowercase character? .db chr + 'A' - 'a' .else .db chr .endif .loop .fclose fhnd ; Close our file handle.
I personally find that rather messy. Here's the new version, using a variety of functions from the 'File Operations' plugin I've been writing:
fhnd = fopen("test.txt", r) #while !feof(fhnd) chr = freadbyte(fhnd) .if chr >= 'a' && data <= 'z' .db chr + 'A' - 'a' .else .db chr .endif #loop fclose(fhnd)
I find that a lot more readable.
An extreme example is the generation of trig tables. Brass 1 uses a series of directives to try and make this easier.
.dbsin angles_in_circle, amplitude_of_wave, start_angle, end_angle, angle_step, DC_offset
Remembering that is not exactly what I'd call easy. If you saw the line of code:
.dbsin 256, 127, 0, 63, 1, 32
...what would you think it did? You'd have to consult the manual, something I'm strongly opposed to. However, this code, which compiles under Brass 2, should be much clearer:
#for theta = 0, theta < 360, ++theta .db min(127, round(128 * sin(deg2rad(theta)))) #loop
By registering new plugins at runtime, you can construct an elaborate pair of directives - in this case .function and .endfunction - to allow users to declare their own.
_PutS = $450A .function bcall(label) rst $28 .dw label .endfunction bcall(_PutS)
You can return values the BASIC way;
.function slow_mul(op1, op2) slow_mul = 0 .rept abs(op1) .if sign(op1) == 1 slow_mul += op2 .else slow_mul -= op2 .endif .loop .endfunction .echo slow_mul(log(100, 10), slow_mul(5, 4))
I had a thought (as you do) that it would be interesting to see how well a TI game would run on the Sega Master System. After all, they share the CPU, albeit at ~3.5MHz on the SMS.
However, there are some other differences...
- Completely different video hardware.
- Completely different input hardware.
- 8KB RAM rather than 32KB RAM.
- No TIOS.
The first problem was the easiest to conquer. The SMS has a background layer, broken up into 8×8 tiles. If I wrote a 12×8 pattern of tiles onto the SMS background layer, and modified the tile data in my own implementation of _grBufCpy routine, I could simulate the TI's bitmapped LCD display (programs using direct LCD control would not be possible).
You can only dump so much data to the VRAM during the active display - it is much safer to only write to the VRAM outside of the active display period. I can give myself a lot more of this by switching off the display above and below the small 96×64 window I'll be rendering to; it's enough to perform two blocks, the left half of the display in one frame, the right in the next.
As for the input, that's not so bad. Writing my own _getK which returned TI-like codes for the 6 SMS buttons (Up, Down, Left, Right, 1 and 2) was fine, but games that used direct input were a bit stuck. I resolved this by writing an Out1 and In1 function that has to be called and simulates the TI keypad hardware, mapping Up/Down/Left/Right/2nd/Alpha to Up/Down/Left/Right/1/2.
The RAM issue can't be resolved easily. Copying some chunks of code to RAM (for self-modifying reasons) was necessary in some cases. As for the lack of the TIOS, there's no option but to write my own implementation of missing functions or dummy functions that don't do anything.
Even with the above, it's still not perfect. If I leave the object code in Gemini, the graphics are corrupted after a couple of seconds of play. I think the stack is overwriting some of the code I've copied to RAM.
No enemies make it a pretty bad 'game', but I thought it was an entertaining experiment.